When we have a web application in production, there are times where some things go wrong and we have errors. By default we will get the “Yellow Screen of Death”. When the application runs in localhost, a lot of information comes up that is useful to find and squash the bug. But when our application runs in production a more vague message appears, only to indicate an error. This default behavior can be controlled with a few tweaks in the web.config file, especially the <customErrors> tag.
You should always set the mode attribute to RemoteOnly, so that you get the asp.net error page with all the debugging info locally, and in production you show an error page. The redirectMode can be set as ResponseRedirect and as ResponseRewrite. The first value will simply redirect the browser and load the error.aspx page. The ResponseRewrite value will load the error.aspx page but the url will not change. That behavior show that your application is a bit more professional.
<customErrors mode="RemoteOnly" redirectMode="ResponseRewrite" defaultRedirect="error.aspx"> </customErrors>
<customErrors mode="RemoteOnly" redirectMode="ResponseRewrite" defaultRedirect="error.aspx"> </customErrors>
Also, you could set different pages to load for a specific error code. If you want to handle these errors more efficiently, you could read this article: Handling redirections, missing pages and server errors in asp.net.
Hiding the error from the website’s visitors is one step. The next step is to log the error and save it to the database. Keeping an errorlog is really useful. Let’s create then the database table errorlog(id, logdate, url, errormessage, innerexception, errorsource, errorstacktrace, errortargetsite). For every error you catch, you could write down this info with the LogError(ByVal ex As Exception) function. The Exception object has useful information that we need.
Sub LogError(ByVal ex As Exception) Dim myComm As New SqlCommand("INSERT INTO errorlog (logdate, url, errormessage, innerexception, errorsource, errorstacktrace, errortargetsite) VALUES (@logdate, @Url, @ErrorMessage, @InnerException, @ErrorSource, @ErrorStackTrace, @ErrorTargetSite)", myConn) myComm.Parameters.Add(New SqlParameter("@logdate", SqlDbType.DateTime)).Value = Now().ToUniversalTime() myComm.Parameters.Add(New SqlParameter("@Url", SqlDbType.NVarChar)).Value = System.Web.HttpContext.Current.Request.Url.AbsoluteUri() myComm.Parameters.Add(New SqlParameter("@ErrorMessage", SqlDbType.NVarChar)).Value = ex.Message If (ex.InnerException IsNot Nothing) Then myComm.Parameters.Add(New SqlParameter("@InnerException", SqlDbType.NVarChar)).Value = ex.InnerException.ToString myComm.Parameters.Add(New SqlParameter("@ErrorSource", SqlDbType.NVarChar)).Value = ex.Source myComm.Parameters.Add(New SqlParameter("@ErrorStackTrace", SqlDbType.NVarChar)).Value = ex.StackTrace myComm.Parameters.Add(New SqlParameter("@ErrorTargetSite", SqlDbType.NVarChar)).Value = ex.TargetSite.ToString() myComm.ExecuteNonQuery() myComm.Dispose() End Sub
We should use this function in every Try…Catch statement and log all the errors our application generates. For errors we haven’t catched, we could take advantage the Application_Error(…) event in the Web.config. There, the Server.LastError is the exception object of the unhandled error.
Sub Application_Error(ByVal sender As Object, ByVal e As EventArgs) LogError(Server.GetLastError) End Sub
The above case is really helpful, but not all errors can be handled that way. There are times that the server return a 500 internal server error. In that case, the above approach will not handle and log the error. This is the time where we have to debug our application on the production server. In that case we need to change a few things in the web.config file.
For IIS 6 you need to set the below:
<configuration> <system.web> <customErrors mode="Off"/> <compilation debug="true"/> </system.web> </configuration>
For IIS 7 you need to set the below:
<configuration> <system.webServer> <httpErrors errorMode="Detailed" /> <asp scriptErrorSentToBrowser="true"/> </system.webServer> <system.web> <customErrors mode="Off"/> <compilation debug="true"/> </system.web> </configuration>
With the above approach you will get a more detailed HTML error code, and in some cases a asp.net error code which can be used to Google it and find out what’s wrong about our web application.
Note: The featured image of this article is from a comic strip from a greek technology blog, iTuts.gr. Here is the actual comic strip, translated in english. One of the characters is a developer, the other one is a designer.
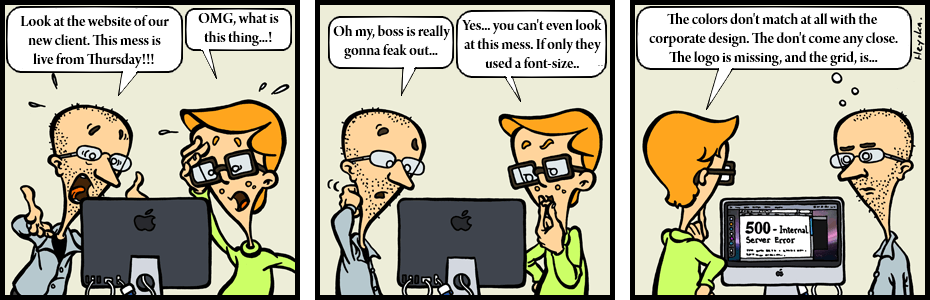
Leave a Reply